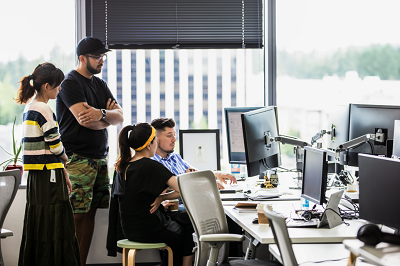
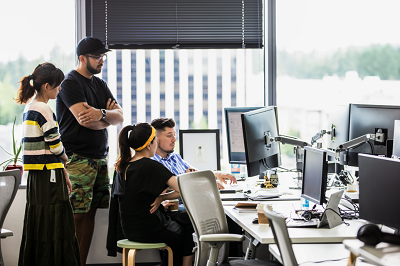
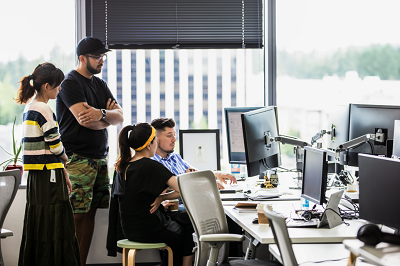
Performance implications of default struct equality in C#
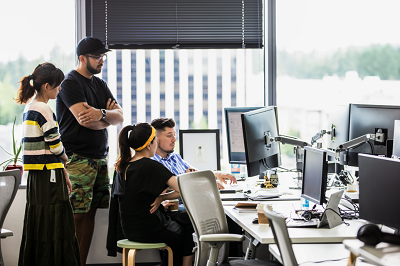
The performance characteristics of async methods in C#
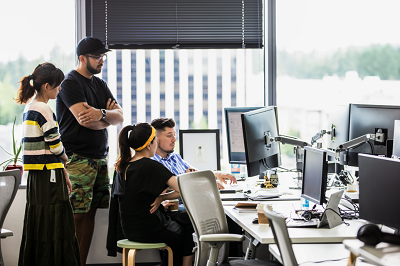
Extending the async methods in C#
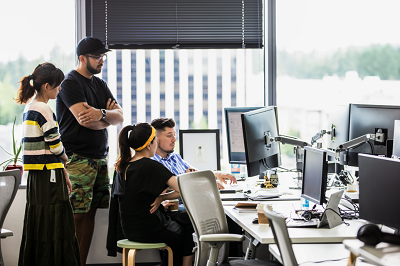
Managed object internals, Part 4. Fields layout
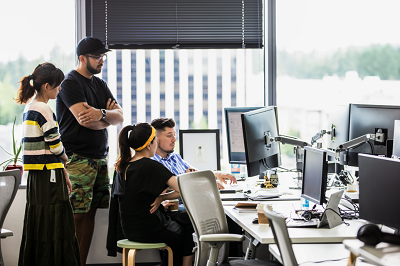
Managed object internals, Part 3. The layout of a managed array
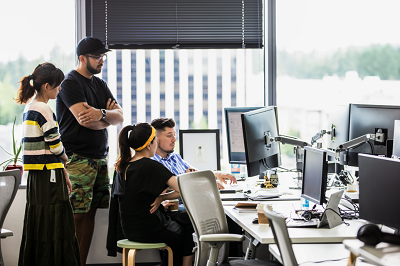
Managed object internals, Part 2. Object header layout and the cost of locking
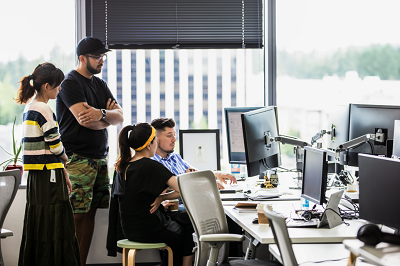
Managed object internals, Part 1. The layout
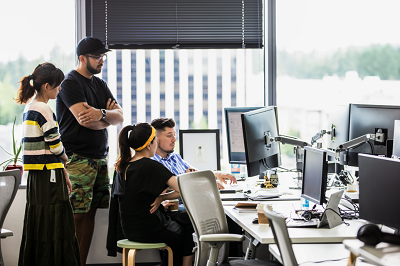
To box or not to Box? That is the question!
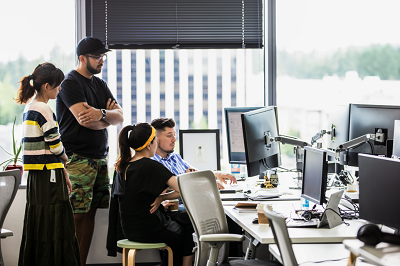