Data Structures: Creating Isolated Storage Files and Directories
In how to use an isolated storage to store a list that can then be used when your project tombstones or you need to save information. In this example, we will take a look at the file structure formed as well as why the file looks the way it does. Isolated storage is the only way you can store information on the phone. If you are thinking about build a Windows Phone Application for a loved one at Christmas or other Holiday gifts, then you will need to store data to make your game or app more enjoyable to use.
To start our exploration, follow the link:
https://msdn.microsoft.com/en-us/library/3ak841sy(v=VS.100).aspx
Use this sample code (from the URL: https://msdn.microsoft.com/en-us/library/6h2ws3ft.aspx )
The code ran on my machine, see section: Working with the code below if you are having difficulties:
using System; using System.IO; using System.IO.IsolatedStorage; public class CreatingFilesDirectories { public static void Main() { // Get a new isolated store for this user, domain, and assembly. // Put the store into an IsolatedStorageFile object. IsolatedStorageFile isoStore = IsolatedStorageFile.GetStore(IsolatedStorageScope.User | IsolatedStorageScope.Domain | IsolatedStorageScope.Assembly, null, null); // This code creates a few different directories. isoStore.CreateDirectory("TopLevelDirectory"); isoStore.CreateDirectory("TopLevelDirectory/SecondLevel"); // This code creates two new directories, one inside the other. isoStore.CreateDirectory("AnotherTopLevelDirectory/InsideDirectory"); // This file is placed in the root. IsolatedStorageFileStream isoStream1 = new IsolatedStorageFileStream("InTheRoot.txt", FileMode.Create, isoStore); Console.WriteLine("Created a new file in the root."); isoStream1.Close(); // This file is placed in the InsideDirectory. IsolatedStorageFileStream isoStream2 = new IsolatedStorageFileStream("AnotherTopLevelDirectory/InsideDirectory/HereIAm.txt", FileMode.Create, isoStore); isoStream2.Close(); Console.WriteLine("Created a new file in the InsideDirectory."); } // End of Main. }
Output:
Working with the code
If you only have the Visual Studio Windows Phone Express on your system, then you will need to follow the instructions that I gave in my earlier blog, where I describe how to create a “console” application using Notepad and the Visual Studio Command Prompt. If you are using the C# Express 2010, which differs slightly from the Visual Studio Windows Phone Express, the Visual Studio Phone Express does not have a console project included in the “New Project” templates. In that case it is just as fast to use the notepad, cut and paste the code using these instructions:
-
- Data Structures in Game Design: List <T> part 1 of many or a few
- If you haven’t worked through that blog, please do so
Discussion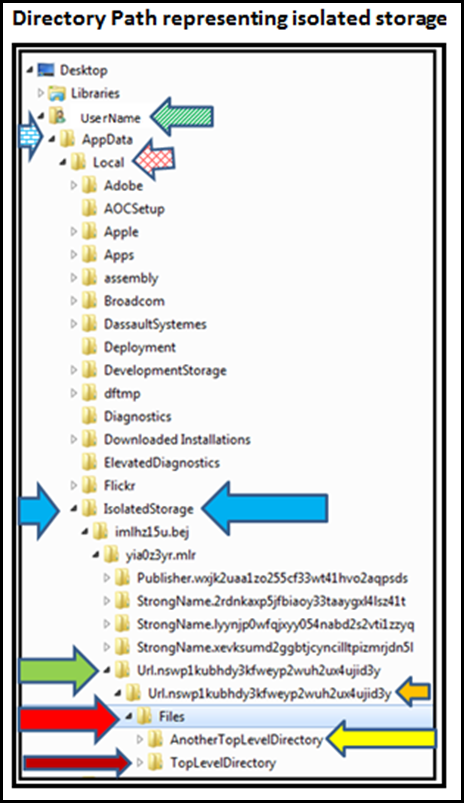
Once you have compiled and run your application, where are the two text files that were created?
- The comments said that the “Top Level Directory” is at the root.
-
-
- Take a look at your “root” directory as it is commonly called, C:\
- Ummm, the directory isn’t there. Where is it?
-
Keep in mind that isolated storage is for the user. Maybe it is under your username! No, not there.
If you do a search on the C:\ drive, at the “root” of the harddrive, then you will find that your files are located at, the path won’t be exactly the same, but the path will be similar and long, The file directory is shown from my machine in the figure.
Why is this?
With isolated storage, your code no longer needs unique paths to specify safe locations in the file system, and data is protected from other applications that only have isolated storage access.
Hard-coded information that indicates where an application's storage area is located is unnecessary.
On the Windows Phone 7, isolated storage is used exclusively as there is no directory structure unlike there is on Window 7. The same way for XBox 360 (and there by Kinect), Xbox and Windows Phone operating systems are very similar.
Next:
How do you manipulate data going into isolated storage, how do you add and remove data moving in and out of the isolated storage?